Introduction
In Magento 2, retrieving order information is a common requirement for developers.
This comprehensive guide explores four different methods to fetch order details using Order ID, comparing their advantages, disadvantages, and best practices.
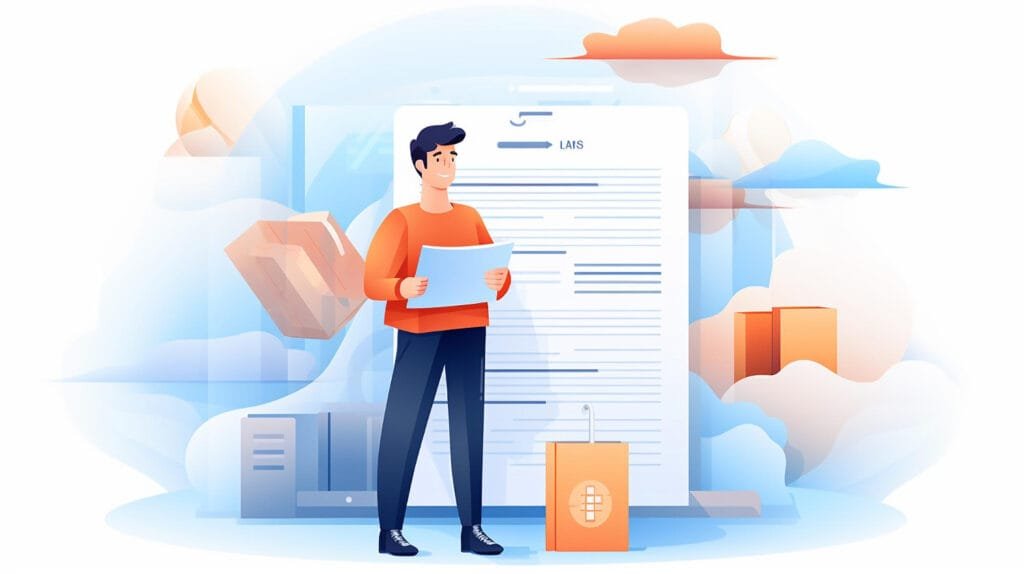
Order Load By Order ID
1. Repository Pattern (Recommended)
The Repository Pattern is Magento 2’s preferred approach for data retrieval. It provides a clean interface between domain models and data mapping layers, ensuring consistent data access and better maintainability.
<?php
namespace Vendor\Module\Block\OrderLoad;
use Magento\Framework\Exception\NoSuchEntityException;
use Magento\Sales\Api\OrderRepositoryInterface;
use Magento\Sales\Api\Data\OrderInterface;
class FetchOrderData extends \Magento\Framework\View\Element\Template
{
/**
* @var OrderRepositoryInterface
*/
private $orderRepository;
/**
* Constructor
*
* @param \Magento\Framework\View\Element\Template\Context $context
* @param \Magento\Sales\Api\OrderRepositoryInterface $orderRepository
* @param array $data
*/
public function __construct(
\Magento\Framework\View\Element\Template\Context $context,
OrderRepositoryInterface $orderRepository,
array $data = []
) {
parent::__construct($context, $data);
$this->orderRepository = $orderRepository;
}
/**
* Get order by ID using Repository Pattern
*
* @param int $orderId
* @return \Magento\Sales\Api\Data\OrderInterface
* @throws NoSuchEntityException
*/
public function getOrderById($orderId)
{
try {
return $this->orderRepository->get($orderId);
} catch (NoSuchEntityException $e) {
throw new NoSuchEntityException(
__('The order with ID "%1" does not exist.', $orderId)
);
}
}
}
// In your phtml file
$orderId = 1;
$order = $block->getOrderById($orderId);
2. Using Object Manager
Direct use of Object Manager, while flexible, is discouraged in production code as it bypasses dependency injection.
<?php
$orderId = 1;
// Using object manager
$objectManager = \Magento\Framework\App\ObjectManager::getInstance();
$order = $objectManager->create('\Magento\Sales\Model\OrderRepository')->get($orderId);
Order Load By Order Increment ID
1. Factory Method
The Factory Method creates instances of order models dynamically, providing flexibility in object creation.
<?php
namespace Vendor\Module\Block\OrderLoad;
use Magento\Framework\Exception\LocalizedException;
use Magento\Sales\Api\Data\OrderInterface;
use Magento\Sales\Model\OrderFactory;
class FetchOrderData extends \Magento\Framework\View\Element\Template
{
/**
* @var OrderFactory
*/
private $orderFactory;
/**
* Constructor
*
* @param \Magento\Framework\View\Element\Template\Context $context
* @param \Magento\Sales\Model\OrderFactory $orderFactory
* @param array $data
*/
public function __construct(
\Magento\Framework\View\Element\Template\Context $context,
OrderFactory $orderFactory,
array $data = []
) {
parent::__construct($context, $data);
$this->orderFactory = $orderFactory;
}
/**
* Get order by increment_id
*
* @param int $incrementId
* @return \Magento\Sales\Api\Data\OrderInterface
* @throws LocalizedException
*/
public function getOrderByIncrementId($incrementId)
{
try {
$order = $this->orderFactory->create()->loadByIncrementId($incrementId);
if (!$order->getId()) {
throw new LocalizedException(__('Order not found'));
}
return $order;
} catch (\Exception $e) {
throw new LocalizedException(
__('Error loading product: %1', $e->getMessage())
);
}
}
}
// In your phtml file
$orderIncrementId = "000000001";
$order = $block->getOrderByIncrementId($orderIncrementId);
2. Object / Model Method
The traditional model approach using direct model loading.
<?php
namespace Vendor\Module\Block\OrderLoad;
use Magento\Framework\Exception\LocalizedException;
use Magento\Sales\Api\Data\OrderInterface;
class FetchOrderData extends \Magento\Framework\View\Element\Template
{
/**
* @var OrderInterface
*/
private $order;
/**
* Constructor
*
* @param \Magento\Framework\View\Element\Template\Context $context
* @param \Magento\Sales\Api\Data\OrderInterface $order
* @param array $data
*/
public function __construct(
\Magento\Framework\View\Element\Template\Context $context,
OrderInterface $order,
array $data = []
) {
parent::__construct($context, $data);
$this->order = $order;
}
/**
* Get order by increment_id
*
* @param int $incrementId
* @return \Magento\Sales\Api\Data\OrderInterface
* @throws LocalizedException
*/
public function getOrderByIncrementId($incrementId)
{
try {
$order = $this->orderinterface->loadByIncrementId($orderIncrementId);
if (!$order->getId()) {
throw new LocalizedException(__('Order not found'));
}
return $order;
} catch (\Exception $e) {
throw new LocalizedException(
__('Error loading order: %1', $e->getMessage())
);
}
}
}
// In your phtml file
$orderIncrementId = "000000001";
$order = $block->getOrderByIncrementId($orderIncrementId);
Get Order Information

1. Display all details of specific order id
<?php
echo "<pre>";
print_r($order->debug());
2. Get Order Information
<?php
$orderData = [];
$orderData = [
"order_id" => $order->getEntityId(),
"increment_id" => $order->getIncrementId(),
"customer_email" => $order->getCustomerEmail(),
"order_state" => $order->getState(),
"order_status" => $order->getStatus(),
"order_total" => $order->getGrandTotal(),
"order_subtotal" => $order->getSubtotal(),
"total_qty_ordered" => $order->getTotalQtyOrdered(),
"order_currency" => $order->getOrderCurrencyCode(),
"store_id" => $order->getStoreId(),
"remote_ip" => $order->getRemoteIp()
];
echo "<pre>";
print_r($orderData);
3. Get Customer Information from Order
<?php
$customerData = [];
$customerData = [
"guestCustomer" => $order->getCustomerIsGuest(),
"customer_id" => $order->getCustomerId(),
"email" => $order->getCustomerEmail,
"groupId " => $order->getCustomerGroupId(),
"firstname" => $order->getCustomerFirstname(),
"middlename" => $order->getCustomerMiddlename(),
"lastname" => $order->getCustomerLastname(),
"prefix" => $order->getCustomerPrefix(),
"suffix" => $order->getCustomerSuffix(),
"dob" => $order->getCustomerDob(),
"taxvat" => $order->getCustomerTaxvat(),
];
echo "<pre>";
print_r($customerData);
4. Get Order Items
<?php
$orderItems = $order->getAllItems();
$items = [];
foreach ($orderItems as $item) {
$items [] = [
"item_id" => $item->getItemId(),
"order_id" => $item->getOrderId(),
"parent_item_id" => $item->getParentItemId(),
"quote_item_id" => $item->getQuoteItemId(),
"store_id" => $item->getStoreId(),
"product_id" => $item->getProductId(),
"sku" => $item->getSku(),
"name" => $item->getName(),
"product_type" => $item->getProductType(),
"is_virtual" => $item->getIsVirtual(),
"weight" => $item->getWeight(),
"qty_ordered" => $item->getQtyOrdered(),
"item_price" => $item->getPrice()
];
}
echo "<pre>";
print_r($items);
5. Get Order Shipping Address
<?php
$orderShippingAddress = [];
/* check order is not virtual */
if (!$order->getIsVirtual()) {
$shippingAddress = $order->getShippingAddress();
$street = $shippingAddress->getStreet();
$orderShippingAddress = [
"firstname" => $shippingAddress->getFirstname(),
"lastname" => $shippingAddress->getLastname(),
"street_1" => isset($street[0])? $street[0]:"",
"street_2" => isset($street[1])? $street[1]:"",
"city" => $shippingAddress->getCity(),
"region_id" => $shippingAddress->getRegionId(),
"region" => $shippingAddress->getRegion(),
"postcode" => $shippingAddress->getPostcode(),
"country_id" => $shippingAddress->getCountryId(),
];
}
echo "<pre>";
print_r($orderShippingAddress);
7. Get Order Billing Address
<?php
$orderBillingAddress = [];
$billingAddress = $order->getBillingAddress();
$street = $billingAddress->getStreet();
$orderBillingAddress = [
"firstname" => $billingAddress->getFirstname(),
"lastname" => $billingAddress->getLastname(),
"street_1" => isset($street[0])? $street[0]:"",
"street_2" => isset($street[1])? $street[1]:"",
"city" => $billingAddress->getCity(),
"region_id" => $billingAddress->getRegionId(),
"region" => $billingAddress->getRegion(),
"postcode" => $billingAddress->getPostcode(),
"country_id" => $billingAddress->getCountryId(),
];
echo "<pre>";
print_r($orderBillingAddress);
8. Get Order Shipping Information
<?php
$shippingData = [];
$shippingData = [
"method_code" => $order->getShippingMethod(),
"method_title" => $order->getShippingDescription(),
"shipping_amount" => $order->getShippingAmount(),
"shipping_discount_amount" => $order->getShippingDiscountAmount(),
"shipping_tax_amount" => $order->getShippingTaxAmount(),
];
echo "<pre>";
print_r($shippingData);
9. Get Order Payment Information
<?php
$paymentData = [];
$payment = $order->getPayment();
$paymentData = [
"method_code" => $payment->getMethod(),
"method_title" => $payment->getAdditionalInformation()['method_title'],
"amount_paid" => $payment->getAmountPaid(),
"amount_ordered" => $payment->getAmountOrdered(),
];
echo "<pre>";
print_r($paymentData);
Comparison of Methods
Aspect | Repository Pattern | Factory Method | Object Manager | Model Method |
---|---|---|---|---|
Maintainability | High | Medium | Low | Medium |
Testability | Excellent | Good | Poor | Good |
Performance | Optimal | Good | Moderate | Good |
Code Readability | High | Medium | Low | Medium |
Dependency Management | Excellent | Good | Poor | Good |
Error Handling | Built-in | Manual | Manual | Manual |
Scalability | High | Medium | Low | Medium |
Future Compatibility | High | Medium | Low | Medium |
Best Practice Recommendations
Conclusion
The Repository Pattern is the most robust and recommended method for retrieving order information in Magento 2.
It provides the best balance of maintainability, testability, and performance while following Magento’s best practices and architectural principles.
Key Takeaways
- Repository Pattern is the recommended approach
- Always implement proper error handling
- Follow Magento 2 coding standards
- Use dependency injection
- Avoid Object Manager in production code
Security Considerations
- Validate order IDs
- Implement proper access controls
- Sanitize input data
- Use proper exception handling
- Follow Magento security best practices
Performance Optimization Tips
- Use collections for multiple orders
- Implement caching where appropriate
- Optimize database queries
- Use lazy loading when possible
- Implement proper indexing
I hope this tutorial useful for you. Please add comment below if you have any query or doubt.
Thank you for reading this article.