Introduction
In this tutorial, Today I will explain to how to use ViewModel in Magento 2.
View Models are a powerful feature in Magento 2 that provide a cleaner way to deliver business logic to templates. Introduced in Magento 2.2, View Models solve many limitations of traditional block classes and promote better separation of concerns. In this tutorial, we’ll explore how to implement View Models effectively using practical examples.
What are View Models?
View Models are simple PHP classes that implement the \Magento\Framework\View\Element\Block\ArgumentInterface
interface. They provide a way to add business logic to templates without extending the \Magento\Framework\View\Element\Template
class, resulting in cleaner, more maintainable code.
Advantages of View Models
Practical Implementation
Let’s create a View Model in our MageDip_Helloworld
module to display customer information.
Let’s assume that you have already created custom module, if you did not create, you can create simple module by this way.
Step 1: Create the View Model Class
File: app/code/MageDip/Helloworld/ViewModel/ExampleViewModel.php
<?php
namespace MageDip\Helloworld\ViewModel;
use Magento\Framework\View\Element\Block\ArgumentInterface;
class ExampleViewModel implements ArgumentInterface
{
/**
* Get title
*
* @return string
*/
public function getTitle(): string
{
return 'Congratulations! This text is coming from View Model.';
}
}
Step 2: Update Layout XML
File: app/code/MageDip/Helloworld/view/frontend/layout/helloworld_index_index.xml
Step 3: Create Template File
File: app/code/MageDip/Helloworld/view/frontend/templates/info.phtml
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<referenceContainer name="content">
<!-- magento custom viewmodel -->
<block class="Magento\Framework\View\Element\Template"
name="customer.info"
template="MageDip_Helloworld::info.phtml">
<arguments>
<argument name="view_model"
xsi:type="object">MageDip\Helloworld\ViewModel\ExampleViewModel</argument>
</arguments>
</block>
</referenceContainer>
</page>
<?php
/** @var \Magento\Framework\View\Element\Template $block */
/** @var \MageDip\Helloworld\ViewModel\ExampleViewModel $viewModel */
$viewModel = $block->getData('view_model');
?>
<div class="view-model-info">
<?php if ($viewModel->getTitle()): ?>
<h2><?= $block->escapeHtml($block->getTitle()) ?></h2>
<?php endif; ?>
</div>
Go to browser and use URL yourdomain.com/helloworld
to see result.
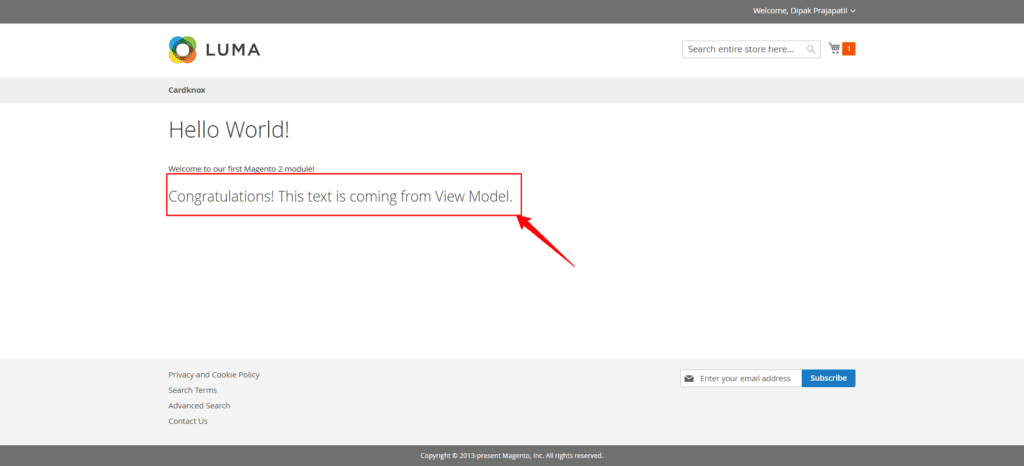
How It Works
- The View Model (
CustomerInfo.php
) contains the business logic and data retrieval methods. - The Layout XML injects the View Model into the block using the
arguments
node. - The Template accesses the View Model using
$block->getData('view_model')
.
Best Practices
- Single Responsibility: Each View Model should focus on a specific functionality.
- Type Hinting: Always use type hints in View Model methods for better code quality.
- Documentation: Add proper PHPDoc blocks for better code readability.
- Dependency Injection: Use constructor injection for dependencies.
- Template Access: Use
getData('view_model')
to access the View Model in templates.
Common Use Cases
- Customer data display
- Product information
- Category listings
- Custom form handling
- API data integration
Comparison with Block Classes
Feature | View Model | Block Class |
---|---|---|
Base Class | Implements Interface | Extends Template |
Responsibility | Business Logic Only | Mixed Concerns |
Testing | Easier | More Complex |
Reusability | High | Lower |
Maintenance | Simpler | More Complex |
Conclusion
View Models represent a significant improvement in Magento 2’s frontend architecture. They provide a cleaner, more maintainable way to deliver business logic to templates compared to traditional block classes. By following the examples and best practices outlined in this tutorial, you can implement View Models effectively in your Magento 2 projects.
Key Takeaways:
- View Models provide better separation of concerns
- They are easier to test and maintain
- They promote code reusability
- They reduce technical debt
- They follow SOLID principles better than block classes
Remember to always consider using View Models instead of extending block classes when adding new functionality to your Magento 2 store.
I hope this tutorial is easy to understand about how to use ViewModel in Magento 2. In case, I missed anything, always feel free to leave a comment in this blog, I’ll get back with proper solution.
Thank you for reading this article. Happy Coding!!!